Integrate Facebook Conversions API in Wix, Using Velo
- Robert Hebert
- Jun 28, 2022
- 3 min read
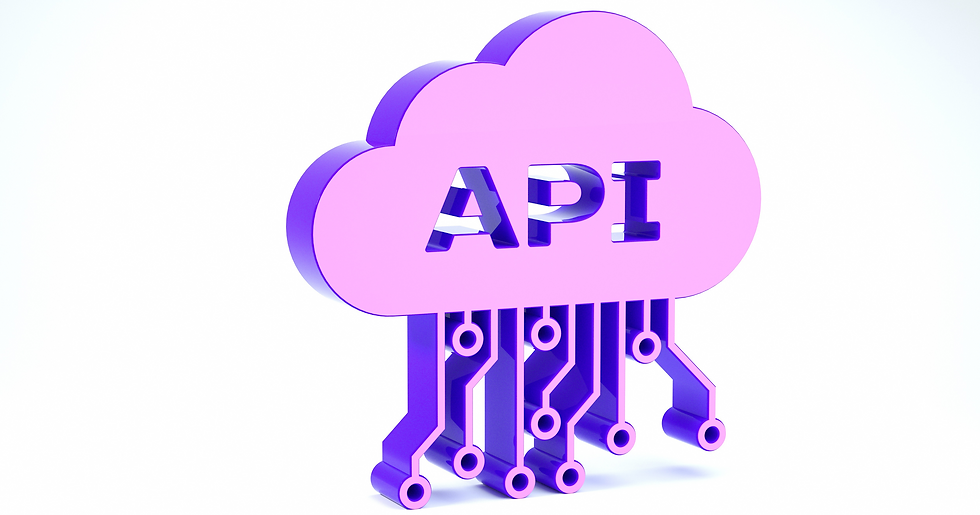
Who is our customer and what problem did they want to solve?
Our customer had previously connected their Facebook Pixel to Wix using the Wix Facebook Pixel & Conversions API Marketing Integration.
This had the clients' website tracking events they had set up in Facebook Business Manager with the pixel, but not with the conversions API.
We were tasked with implementing the Conversions API for as many of the events as we could (View Content, Add to Cart, and Initiate Checkout).
What solution did we provide?
Since the Conversions API is all about sending tracking data from the server rather than the client, we knew that we would need a way to send the event information from the frontend to the backend.
So we decided to make use of Wix HTTP Functions to provide an endpoint for the website to send event tracking requests to have them processed on the backend and sent to Facebook via the Conversions API.
How did we implement the solution?
We implemented a single endpoint called `trackEvent` to accomplish this, using the wix-fetch module as well as the wix-secrets-backend module to store & retrieve the Pixel Id and Access Token needed to successfully call the Conversions API. When called from the frontend this endpoint would take the data sent to it and format it to send to Facebook.
After this endpoint was implemented, we add code to the `masterPage.js` file which is included on every page of a Wix site.
The code parses information about what page the user is currently on and gathers data based on the event and then sends a request to the `trackEvent` endpoint to have it sent over to Facebook's Conversion API.
Check out the code we used below!
import { getSecret } from "wix-secrets-backend";
import { fetch } from "wix-fetch";
import { ok, badRequest } from "wix-http-functions";
const baseURL = "https://graph.facebook.com/v12.0/<PIXEL_ID>/events";
export async function post_trackEvent(request) {
const pixelId = await getSecret("FbPixelId");
const accessToken = await getSecret("FbCAPIAccessToken");
const URL =
baseURL.replace("<PIXEL_ID>", pixelId) + `?access_token=${accessToken}`;
const today = new Date();
const current_timestamp = Math.floor(today / 1000);
const ipAddress = request.ip;
const body = await request.body.json().then((json) => json);
const userData = {
client_ip_address: ipAddress,
client_user_agent: body.userAgent,
};
let customData;
if (body.content) {
customData = {
content_category: "All Products",
content_name: body.content.name,
value: body.content.value,
quantity: body.content.quantity,
content_type: "product",
currency: "usd",
content_ids: [body.content.id],
};
}
if (body.eventName === "InitiateCheckout") {
customData = {
content_category: "All Products",
contents: body.content.contents,
value: body.content.value,
content_type: "product",
currency: "usd",
content_ids: body.content.content_ids,
num_items: body.content.num_items,
};
}
const reqBody = {
event_name: body.eventName,
event_time: current_timestamp,
event_source_url: body.sourceUrl,
action_source: "website",
user_data: userData,
};
if (customData) {
reqBody.custom_data = customData;
}
const headers = {
"Content-Type": "application/json",
};
fetch(URL, {
method: "POST",
headers,
body: JSON.stringify({ data: [reqBody] }),
})
.then((res) => res.json())
.then((json) => {
console.log(json);
})
.catch((err) => {
console.log("Error: ", err);
});
const response = {
headers: {
"Content-Type": "application/json",
},
};
try {
response.body = {
response: {
pixelId,
},
};
return ok(response);
} catch (err) {
response.body = {
error: err,
};
return badRequest(response);
}
}
Have any questions? Contact us at 225-250-1888 or email robert@roberthebertmedia.com.
About our company
RHM specializes in helping businesses of all sizes and across all industries achieve their digital and web marketing needs. Whether it's designing a new website, building an app, performing custom development, or running Google Ads, our goal is to showcase how you are the best at what you do and help people connect with you. Contact us at 225-250-1888 to get started!
Comments